Flower
./** * Flower.java * @author Jose M Vidal* Created on Jan 24, 2012 * The skeleton code for building a drawing application */ import java.awt.Color; import java.awt.Graphics; import java.awt.Graphics2D; import javax.swing.JFrame; import javax.swing.JPanel; public class Flower extends JPanel { public void paintComponent(Graphics g){ final int centerX = 300; //the centerX and Y coordinates of our flower. final int centerY = 300; final int ovalWidth = 100; //the width of the oval. A petal is an oval. Graphics2D g2 = (Graphics2D) g; //TODO: Your code goes IN HERE (not in main), ALL OF IT. Replace the code below. //draw one oval of length 300pixels from center to edge g2.setColor(Color.black); g2.fillOval(centerX, centerY - ovalWidth / 2, 300, ovalWidth); //rotate the coordinate system by 15 degrees, around the centerX/Y. g2.rotate(Math.toRadians(15),centerX,centerY); //draw a second oval g2.fillOval(centerX, centerY - ovalWidth / 2, 300, ovalWidth); g2.rotate(Math.toRadians(15),centerX,centerY); //draw a third oval g2.fillOval(centerX, centerY - ovalWidth / 2, 300, ovalWidth); g2.rotate(Math.toRadians(15),centerX,centerY); } // You do NOT need to change anything in main(). Leave it as is. public static void main(String[] args) { JFrame frame = new JFrame("Window"); //frame is the window frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); Flower panel = new Flower(); //panel is the graphics area where we can draw frame.add(panel); //put the panel inside the window frame.setSize(640,640); //set the window size to 640x640 pixels frame.setVisible(true); } }
When you run that program it will pop up a window which will look like the one pictured here on the right in Figure 1. As you can see, this program draws 3 black petals. Each petal is actually just a filled-in oval, which we draw using the
fillOval()
method. We then rotate the coordinate system by 15 degrees around the center using rotate()
. Thus, when we draw the second oval it appears rotated down, as a petal in a flower might.For this lab you will modify that code so that your new program instead draws a flower like the one shown in Figure 2. You will do this by replacing the code inside the
paintComponent
method (you do not need to change anything in main
). Replace the code in there that draws the 3 ovals, with a loop that draws 275 ovals. Follow these steps:- Change the rotation from 15 degrees to one ϕ of a circle, which is 137.5 degrees. See the video below for why this is the number Nature uses.
- Add a loop that draws 275 successive ovals, each one 137.5 degrees from the previous one.
- The first oval should be of a length 300 (as the ones in the code above are), the next one 299, the next one 288, and so on.
- Make the color of the ovals alternate:
Color.blue
thenColor.cyan
then back toColor.blue
and so on. For the first 250 petals. - The last 25 petals are all
Color.yellow
. - Finally, to get the black outline on the petals, simply draw a black oval that is an extra 2 pixels in width and length, then draw the colored oval as usual.
Hint: You do not need to read the documentation to find more
Graphics
operations. All you need is: setColor
, fillOval
and rotate
, as shown in the sample code. Do read the documentation for those 3 methods.This lab was inspired by Vi's video below, but note that she draws her flowers starting with the smallest petal, while your code starts with the biggest petal and proceeds to smaller and smaller petals. We do this because it is much easier to draw over something than it is to draw behind something.
If you are like me, you can't help but play around by changing some of the numbers to see what comes up. Below are some of the flowers I made by just changing the code around a bit.
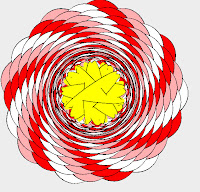
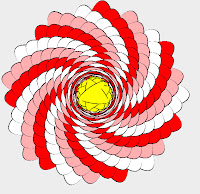
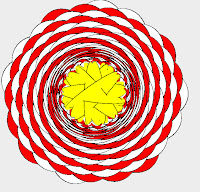

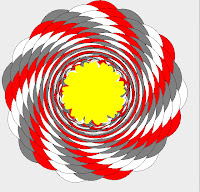
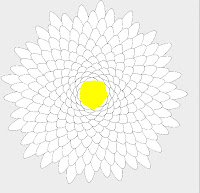
If you make some pretty ones that you want to share, just email them to me or post them on the web somewhere and let me know. I will add them to our flower gallery right here! BTW, here is how to take-a-screenshot.org
No comments:
Post a Comment