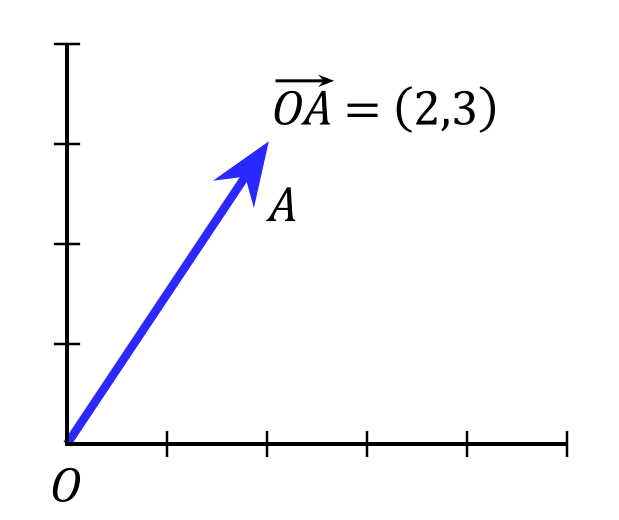
A vector in 2-dimensional space, as used in math an physics, is just a pair of numbers: delta-x and delta-y. We call these numbers the components of the vector. Vectors are drawn as arrows. For example, the vector in the figure has a delta-x of 2, and a delta-y of 3. In physics, vectors represent forces. Adding two vectors represents placing those two forces on an object. The resulting force is just the addition of the two vectors. You can add two vectors by simply adding their delta-x and delta-y values. That is, the new delta-x is the sum of the delta-x of the two other vectors.
More formally, for vectors
v1
and v2
with components v1.dx
, v1.dy
and v2.dx
, v2.dy
, we can define various vector operations:- Vector addition:
v1 + v2 = v3
is implemented asv3.dx = v1.dx + v2.dx
andv3.dy = v1.dy + v2.dy
- Vector subtraction:
v1 - v2 = v3
is implemented asv3.dx = v1.dx - v2.dx
andv3.dy = v1.dy - v2.dy
- Vector scalar multiplication:
v1 * c = v2
wherec
is a constant is implemented asv2.dx = v1.dx * c
andv2.dy = v1.dy * c
- Vector magnitude of
v1
is a number equal to the square root ofv1.dx*v1.dx + v1.dy*v1.dy
. - A vector
v1
can be normalized by settingv1.dx = v1.dx / v1.magnitude
andv1.dy = v1.dy / v1.magnitude
Vector
class that has the following data members:- dx, a double
- dy, a double
- A constructor that takes as argument two numbers which will become the delta-x and delta-y
public void addVector(Vector otherVector)
which addsotherVector
to this vectorpublic void subtractVector(Vector otherVector)
which subtractsotherVector
from this vectorpublic void multiply(int a)
does a scalar multiplication of this vector bya
public double getMagnitude()
returns the magnitude of this vector.public void normalize()
normalizes this vector.public String toString()
returns a printable version of the vector.
You must also implement a main
function that tests all your methods to make sure they are correct.
One very important rule you must follow is Don't Repeat Yourself (DRY) which means that:
“Every piece of knowledge must have a single, unambiguous, authoritative representation within a system ”In other words, any value that can be calculated from data member values must be calculated from data members values. This homework is due Tuesday, October 6 @ noon.